In this article, we’ll share our experience migrating a website from a traditional dotCMS setup to a modern Universal CMS approach using dotCMS and Next.js.
This project was particularly exciting because we used dotCMS’s official libraries while they were still in the alpha stage. The migration allowed us to improve performance, scalability, and development flexibility.
We’ll walk through our planning process, project structure, key challenges, and lessons learned. If you're considering using dotCMS as a Universal CMS, this guide will help you understand how easy it is and why it's worth it.
Why Did We Migrate?
Before diving into the technical details, let’s explain why we made the switch:
The existing site needed a facelift – Branding modernization required a more up-to-date website.
More flexibility – A Universal CMS separates content management from the front end, allowing for faster iterations.
Universal Editing – The content author will have complete experience with all the required actions.
Better SEO and performance – With Next.js, we could use Server-Side Rendering (SSR) and Static Site Generation (SSG) to improve load times and search engine visibility.
Modern development workflow – Next.js provided a scalable, maintainable, and developer-friendly architecture. Additionally, we strive to align them with modern web development standards, so we chose Tailwind as our styling framework; and NextJS offers easy integration with this.
What is a Universal CMS?
A Universal CMS is a content management system designed to work seamlessly across all technologies and channels**. It provides a unified platform where developers and content editors can do their best work.**
dotCMS bridges the gap between traditional and headless CMSs by offering a single, intuitive editorial interface. This allows content teams to manage content efficiently without constantly relying on developers. It combines powerful visual editing tools with the flexibility developers need, ensuring a smooth and consistent experience for everyone.
With its hybrid-headless approach, a Universal CMS lets developers use any front-end framework while giving content teams the tools to maintain full visual control.
Learn more about Universal CMS and its benefits: Creating a Unified Editor Experience: Universal Visual Editor.
How do these approaches compare?
Traditional CMS: Uses templates and server-side rendering.
Headless CMS: Exposes content via REST and GraphQL APIs.
Universal CMS: Combines the best of both, offering API-driven flexibility with built-in visual editing tools.
Migration Planning
Before jumping into development, we defined the key requirements for the new website:
Support for multiple content types – Blogs, News, Podcasts, Partners, etc.
SEO-friendly – With SSR, dynamic metadata, and AI-crawlable structured data to enhance discoverability.
Fast load times – Using Static Site Generation (SSG) for static pages.
Efficient API usage – Combining REST and GraphQL for optimal performance.
This approach ensures that the website is not only optimized for traditional search engines but also for AI-driven content discovery. Learn more about AI Crawler.
Technical Stack
dotCMS – Universal CMS → Content management
GraphQL & REST → APIs to fetch content
Next.js → Front-end framework
Vercel → Deployment
Project Structure
Our Next.js project is organized as follows:
.
└── src
├── app
│ ├── [[...slug]]
│ │ └── page.js
│ ├── blog
│ │ └── [blogSlug]
│ │ └── page.js
│ └── news
│ └── [newSlug]
│ └── page.js
├── components
│ ├── content-types/
│ ├── layout/
│ └── shared/
├── hooks/
└── pages/api <-- API Routes. For more information check
We dynamically render content based on the URL and content type retrieved from dotCMS.
To learn more about NextJS API Routes, visit their documentation.
From Challenges to Success: How We Built a Better Site
Building a high-performing, scalable website with a headless CMS architecture comes with unique challenges: managing different page types, optimizing API calls, improving SEO, and — most importantly — ensuring a seamless experience for both content authors and developers.
In this section, we’ll walk through the key challenges we faced, how we tackled them, and the best practices we implemented to build a faster, more flexible, and future-proof site.
How we manage different page types
Our site needed to support three distinct types of pages, each with different content structures and rendering requirements:
Simple pages: These are static content pages managed in dotCMS, such as /about-us and /partners. They primarily consist of text, images, and links, making them ideal for standard informational content.
Detail pages: These pages follow a predefined template but display dynamic content based on the URL path. For example, URLs like /blog/some-blog or /news/some-news dynamically load content from dotCMS. (For more details, see URL Mapped Content).
Hybrid Pages: These pages combine content from dotCMS with additional hardcoded elements that exist only in Next.js. A common use case is a blog post that includes a "Related Blogs" section, pulling data from dotCMS while also featuring custom sections unique to the front end.
Approach:
We leveraged Next.js routing capabilities to structure our solution efficiently.
For simple pages, we used optional catch-all segments ([[...slug]]) to capture the requested path. With this data, we retrieve the corresponding page content using the dotCMS SDK and render it with the DotcmsLayout component.
We combined Server Components and Client Components, depending on the specific requirements of each page.
Pseudocode Example:
// [[...slug]]/page.js
import { DotCmsClient } from "@dotcms/client";
import { DotLayoutComponent } from "@dotcms/react";
export default async function Page({ searchParams, params }) {
const client = DotCmsClient.init({
dotcmsUrl: DOTCMS_INSTANCE_URL,
authToken: DOTCMS_AUTH_TOKEN,
siteId: DOTCMS_SITE_ID,
});
const requestParams = {
path: params.slug,
language_id: searchParams.language_id,
};
const pageAsset = await client.page.get(requestParams);
// Define which component to render for each piece of content within the page
const componentsMap = { /* Component mappings */ };
return (
<DotLayoutComponent
pageContext={{
pageAsset,
components: componentsMap,
}}
/>
);
}
For detail pages, we couldn’t rely entirely on the optional catch-all solution because these pages required additional data, and their templates differed from those used for simple pages.
To address this, we structured our Next.js app using separate folders for each type of detail page. We implemented the App Router approach in Next.js (Next.js App Router Docs) to ensure flexibility and maintainability.
.
└── src
└── app
├── [[...slug]]
│ └── page.js # Single Pages
├── blog
│ └── [blogSlug]
│ └── page.js # Detail Pages + Custom Sections
└── news
└── [newSlug]
└── page.js # Detail Pages
With this setup:
Simple pages are handled in [[...slug]]/page.js.
Detail pages (e.g., blogs and news) have their own dedicated folders (/blog and /news), where we capture the path segment, build the query using the dotCMS SDK, and apply any additional logic needed.
Rendering Blog Content
We used the BlockEditorRenderer component specifically for blog pages. This allows us to render HTML content from the Block Editor field in dotCMS.
This way, we can edit blog content in dotCMS and render it on our website using only the components provided by the dotCMS SDK. Additionally, this component allows customization of how each content type is rendered, making it perfect for applying your own branding style.
Pseudocode Example:
/* /blog/[...blogSlug]/page.js */
import { DotCmsClient } from "@dotcms/client";
import { BlockEditorRenderer } from "@dotcms/react";
const client = DotCmsClient.init({
dotcmsUrl: DOTCMS_INSTANCE_URL,
authToken: DOTCMS_AUTH_TOKEN,
siteId: DOTCMS_SITE_ID,
});
function BlogDetailPage({ searchParams, params }) {
const requestParams = {
path: "/blog/" + params.slug,
language_id: searchParams.language_id,
};
const { urlContentMap } = await client.page.get(requestParams);
return (
<div className="my-blog-custom-class">
<BlockEditorRenderer blocks={urlContentMap.body} />
...More content
</div>
);
}
export default BlogDetailPage;
For Hybrid pages, we used the dotCMS Content API, which allows us to query only the data we need and render it as required. Hybrid pages refer to content stored in dotCMS that isn’t tied to a specific page but still needs to be displayed dynamically.
This approach allows us to create queries with exactly the data we need and use it to render the required content dynamically.
This approach was especially useful for features like "Related Blogs" on the detail blog post page. Since related blogs aren’t part of the current page but are stored in dotCMS, we needed a way to fetch and display them dynamically.
Pseudocode Example:
/* /blog/[...blogSlug]/page.js */
import { DotCmsClient } from "@dotcms/client";
import { BlockEditorRenderer } from "@dotcms/react";
const client = DotCmsClient.init({
dotcmsUrl: DOTCMS_INSTANCE_URL,
authToken: DOTCMS_AUTH_TOKEN,
siteId: DOTCMS_SITE_ID,
});
async function BlogDetailPage({ searchParams, params }) {
const requestParams = {
path: "/blog/" + params.slug,
language_id: searchParams.language_id,
};
// Fetch the current blog post
const { urlContentMap } = await client.page.get(requestParams);
// Retrieve related blog articles based on the same category
const latestBlog = await client.content
.getCollection("Blog")
.limit(4)
.query((qb) =>
qb
.field("categories")
.equals(urlContentMap.categories[0]?.key || "")
.excludeField("title")
.equals(urlContentMap.title)
.field("showInListing")
.equals("true")
.field("live")
.equals("true")
);
const relatedBlog = latestBlog.contentlets;
return (
<div className="my-blog-custom-class">
<BlockEditorRenderer blocks={urlContentMap.body} />
{/* Additional content */}
<hr />
<h3>Related Blog</h3>
{relatedBlog.map((blog) => (
<MyBlogCard key={blog.identifier} blog={blog} />
))}
</div>
);
}
export default BlogDetailPage;
Boosting Performance with the Right API Strategy
Fetching content dynamically can slow down page loads, especially when making multiple API requests. To improve performance, strategy is essential.
Approach:
We optimized API calls by selecting the right strategy for each page section. In static sections like navigation/related posts, we use GraphQL to take advantage of their cache system. In another section, we opted for REST API. In summary:
GraphQL for complex queries (e.g., fetching related content in detail pages).
REST API for small, frequently used content lists.
By combining REST for efficiency and GraphQL for flexibility, we significantly reduced API response times.GraphQL Example: Fetching the Navigation Menu
For static sections like the navigation menu or related posts, we use GraphQL, taking advantage of its caching system to speed up responses.
Here’s how we fetch the navigation menu using GraphQL:
export const navigationQuery = `{
htmlpageassetCollection(query: "+(conhost:${YOUR_HOST} +live:true") {
title
url
icon
navigation
menuTitle
menuSubtitle
menuSummary
showOnMenu
showOnMenuAsCategory
section
sortOrder
folder {
folderPath
folderTitle
folderDefaultFileType
folderSortOrder
folderName
folderId
folderFileMask
}
redirecturl
}
}`;export const getNavigation = async () => {
const res = await fetch(API_URL, {
method: 'POST',
headers: {
Authorization: `Bearer ${process.env.NEXT_PUBLIC_DOTCMS_AUTH_TOKEN}`,
},
body: JSON.stringify({ query: navQuery })
});
const { data } = await res.json();
return data.htmlpageassetCollection;
};
We use the getNavigation function in SSR (Server-Side Rendering) to fetch the site navigation menu. This approach is fast because of GraphQL caching and Next.js cache memory.
Learn more about the dotCMS GraphQL API.
REST API Example: Fetching Page Data
For simpler, frequently used data, we use the REST API. Our client, @dotcms/client, provides a more developer-friendly way to interact with the dotCMS API.
Here’s an example of how we fetch page data using our client SDK:
import { DotCmsClient } from "@dotcms/client";
const client = DotCmsClient.init({
dotcmsUrl: 'YOUR_DOTCMS_HOST',
authToken: 'YOUR_AUTH_TOKEN',
siteId: 'YOUR_SITE_ID'
});
export const getDotCMSPageAsset = async (requestParams) => {
try {
const dotCMSPageAsset = await client.page.get(requestParams);
return { dotCMSPageAsset };
} catch (error) {
return { error };
}
};
By strategically selecting when to use GraphQL and REST, we improved performance while keeping API requests optimized for different use cases.
Maximizing Speed and Search Visibility
To ensure our pages load quickly and are fully indexable by search engines, we followed these key strategies:
SSR (Server-Side Rendering) for dynamic pages.
SSG (Static Site Generation) for informational pages.
Dynamic meta tags using generateMetadata().
JSON-LD for a linked data format.
Dynamic Meta Tags
Next.js makes it easy to generate meta tags dynamically based on page data. In dotCMS, each page has a section where meta tags are defined. Using this data, we dynamically generate the appropriate metadata in Next.js.
Here’s an example of how we set up metadata in Next.js:
import { getPageData, extractRobotsMeta } from '@/utils';
import { DOTCMS_DEFAULT, DOTCMS_URL } from '@/constants';
export async function generateMetadata({ params, searchParams }) {
const { pageAsset } = await getPageData(params.slug, searchParams);
const { page } = pageAsset;
const canonicalUrl = page.canonicalUrl || page.pageURI;
return {
title: page.pageTitle,
description: page.metaDescription,
keywords: page.metaKeywords,
authors: [{ name: DOTCMS_DEFAULT, url: DOTCMS_URL }],
creator: DOTCMS_DEFAULT,
publisher: DOTCMS_DEFAULT,
openGraph: {
title: page.ogTitle,
description: page.ogDescription,
type: page.ogType,
url: DOTCMS_URL,
siteName: DOTCMS_DEFAULT,
},
robots: extractRobotsMeta(page?.searchEngineIndex),
alternates: {
canonical: canonicalUrl
}
};
}
Learn more about Next.js meta tags.
Managing Media Assets with dotCMS: Images, Videos, and More
dotCMS stored assets externally, and fetching them caused CORS issues and slow loading.
The solution:
We created a custom ImageLoader for Next.js to serve images directly from dotCMS instead of our local environment. Setting this up was simple because dotCMS follows a consistent URL pattern for accessing assets.
With dotCMS, you can retrieve any asset using this format:
dotCMSInstaceURL/dA/dotCMSInstaceURL/dA/{InodeOrIdentifier}
Next.js also makes it easy to define a custom image loader. Here’s how we did it:
const DOTCMS_URL = 'https://dotcms-cloud-instance.com';
const ImageLoader = ({ src, width }) => {
const imagePath = src.includes('/dA/') ? src : `/dA/${src}`; // Ensures correct asset path
return `${DOTCMS_URL}${imagePath}/${width}w?language_id=1`;
};
export default ImageLoader;
In addition to images, we also use a proxy for other assets, such as documents and files. This ensures that all requests for dotCMS assets go through our Next.js application. Here’s how we configured next.config.js:
next.config.js
/** @type {import('next').NextConfig} */
const url = new URL(process.env.NEXT_PUBLIC_DOTCMS_HOST);
const nextConfig = {
async rewrites() {
return {
beforeFiles: [
{
source: '/dA/:path*',
destination: `${url.origin}/dA/:path*`
}
]
};
}
};
module.exports = nextConfig;
With this setup:
Images are served via a custom ImageLoader.
Other assets (e.g., PDFs, videos) are proxied through Next.js to maintain consistent URLs.
This approach improves performance, ensures proper asset loading, and keeps all dotCMS assets accessible in a structured way.
Learn more about dotCMS assets and Next.js custom image loaders.
Final Thoughts and Recommendations
🚀 Migrating from a traditional dotCMS setup to a Universal CMS approach with Next.js was a game-changer. It gave us more flexibility, better performance, and a modern development workflow.
The final result? We move on from this page:
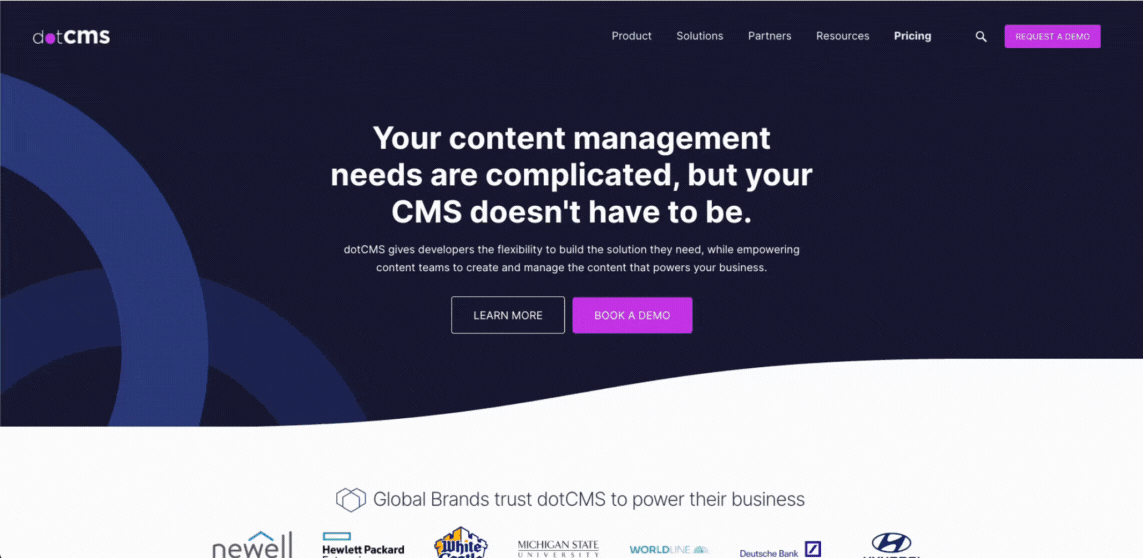
To this modern page:
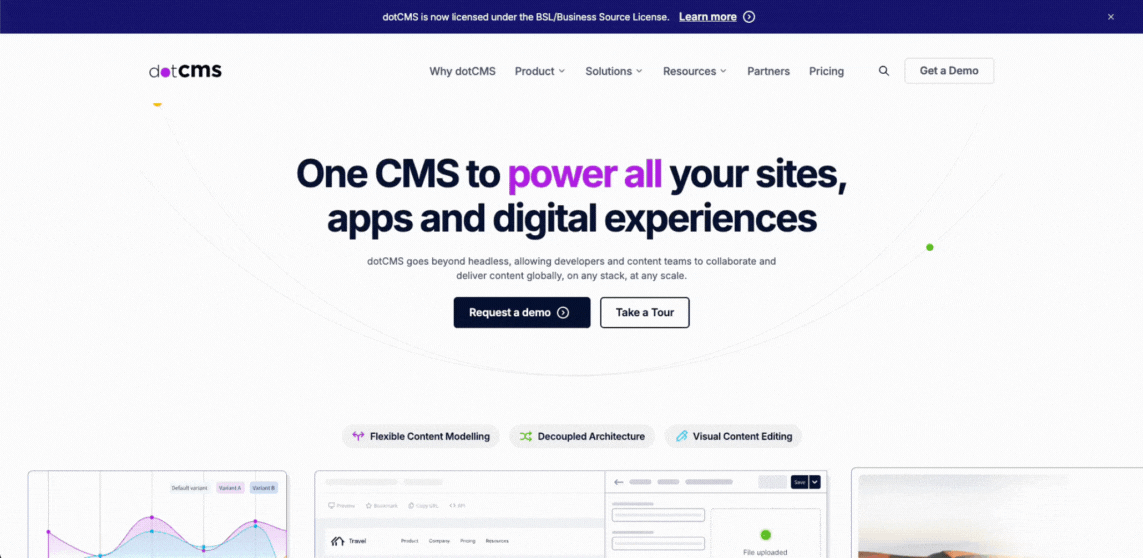
Maintaining the robustness that dotCMS offers, with all the benefits of modern web development.
You can check it out yourself by visiting www.dotcms.com
Lessons Learned & Best Practices
Throughout this migration, we identified key strategies that ensured a smooth transition:
Plan Your Content Model Early – Defining a structured content model in dotCMS helped us avoid reworking later.
Use a Hybrid API Strategy – Combining GraphQL for complex queries and REST for frequently used content improved performance.
Optimize for SEO from the Start – Using SSR, dynamic metadata, and JSON-LD made a significant difference in search visibility.
Leverage Next.js Features – Implementing SSG for static content and ISR (Incremental Static Regeneration) for updates boosted load times
Key Takeaways:
Decoupling content from UI makes maintenance easier.
Next.js + dotCMS is a powerful combination for Universal CMS projects.
Using GraphQL & REST strategically improves performance.
Investing time in SEO and performance pays off in the long run.
💡 If you’re thinking about using dotCMS as a Headless CMS, check out these resources: