JavaScript is everywhere, and its growth shows no signs of slowing down. Every day, new libraries, frameworks, engines, builders, and transpilers emerge — constantly pushing the ecosystem forward.
But why is that? One big reason is that JavaScript is a universal language. In other words, you can write isomorphic code — code that runs seamlessly in both the browser and a server environment. But what does that actually mean?
What Does ‘Universal’ Mean?
In simple words, JavaScript can run on both the server (Node.js) and the client (browser), thanks to V8. This engine takes care of parsing JavaScript, turning it into an Abstract Syntax Tree (AST), and then compiling it into machine code that can be executed.
Since V8 powers both server-side and client-side environments, runtimes like Node.js and Bun can extend its capabilities — making JavaScript fully functional beyond just the browser.
However, just because JavaScript works in a "universal" way, it doesn't mean the code is 100% compatible across environments.
There are differences like file extensions, how we import and export modules, how code is exposed, and how the global variable is accessed, among other things.
In this tutorial, we’ll focus on the differences in file extensions and imports — these will be key to building our SDK and making it accessible to the world.
File Extensions
JavaScript uses various file extensions, and they play a crucial role in how the environment decides to treat the file. Let’s examine the ones we need.
JavaScript Modules (.mjs)
This extension is used for ECMAScript modules (ESM) in JavaScript files. It’s commonly used in modern frameworks such as React, Angular and Astro.
The key difference with .mjs files is that they use import and export for modularity — the modern, standardized way to handle modules in JavaScript.
For example, we have two files: utils.mjs and index.mjs
utils.mjs
export function greet(name) {
return Hello, ${name}!;
}
As you can see in the code above, we can simply export the function. Then, in index.mjs
import { greet } from './utils.mjs'; // Import using ES modules
console.log(greet('Alice')); // Output: Hello, Alice!
We can import the function and use it right away.
This is the new, modern way to work with modules. However, we can also write code using CommonJS, which is more commonly used in the Node.js environment.
CommonJS (.cjs)
This extension stands for CommonJS, the older module system used in Node.js. Before ESM became the standard, CommonJS was the default way to handle modules in JavaScript, especially in Node.js.
The key difference from ESM is that CommonJS uses require and module.exports to import and export modules.
Let’s rewrite the previous example using CommonJS.
utils.cjs
function greet(name) {
return `Hello, ${name}!`;
}
module.exports = { greet };
As you can see in the code above, we use module.exports to export the function. Then, in index.cjs
const { greet } = require('./utils.cjs'); // Import using CommonJS
console.log(greet('Alice')); // Output: Hello, Alice!
Here, we use the require function to import the module. Although this is no longer the standard, many Node.js libraries still rely on CommonJS. To make our project truly universal, we need to ensure compatibility with both module systems.
But does that mean we have to develop the SDK twice — once for .mjs and once for .cjs?
Not quite. That’s where building comes in.
What Does ‘Building’ Mean?
The build process is a step in the development lifecycle where your code gets processed by a builder, so that it’s bundled, minified, transpiled, optimized, and has unused code removed.
The goal is to make your code production-ready, ensuring it’s as lightweight and optimized as possible for faster loading times — and also, to make it universal.
But before we start setting up the build process, let’s go over a few prerequisites to make sure you have everything you need.
Prerequisites
Before we begin, I'll be reusing some code from a previous blog post. I recommend checking it out first for better context.
However, if you’d rather jump right in, you can grab the code from this repo in the client-example folder.
You'll also need Node.js LTS installed. Make sure npm is available, and use your preferred IDE and terminal to follow along.
Installing Rollup.js
Rollup.js is a JavaScript bundler that’s perfect for our task, so we’ll be using it in this tutorial.
Open the root folder of the project I mentioned earlier on your terminal and run the following command:
npm i --save-dev @rollup/plugin-terser @rollup/plugin-typescript @types/node rimraf rollup rollup-plugin-dts tslib rollup-plugin-copy
Breaking It Down
This command installs the following libraries as development dependencies:
@rollup/plugin-terser – Minifies the code for better performance.
@rollup/plugin-typescript – Transpiles TypeScript to JavaScript.
@types/node – Provides TypeScript type definitions for Node.js.
rimraf – Ensures a clean build by deleting previous build files.
rollup – The core bundler we’ll use.
rollup-plugin-dts – Generates .d.ts files for TypeScript support.
tslib – A required peer dependency for Rollup’s TypeScript plugin.
rollup-plugin-copy – Copies package.json into the build output.
With these in place, we’re ready to configure Rollup.
Configuring Rollup
Open your IDE and navigate to your project’s root directory. Create a new file named rollup.config.ts, then add the following content:
import type { InputOptions, OutputOptions, RollupOptions } from 'rollup';
import typescriptPlugin from '@rollup/plugin-typescript';
import terserPlugin from '@rollup/plugin-terser';
import dtsPlugin from 'rollup-plugin-dts';
import copy from 'rollup-plugin-copy';
// Our output path
const outputPath = 'dist/dotcms-client';
// Our common input options, all configs will use these plugins
const commonInputOptions: InputOptions = {
input: 'src/index.ts', // The entry point of our SDK
plugins: [
typescriptPlugin({
tsconfig: './tsconfig.json',
declaration: false, // We'll generate declarations separately
module: 'esnext', // Ensure TypeScript outputs modern JavaScript modules
target: 'es2022' // Using modern JavaScript features
}),
// Copy the package.json to the dist folder
copy({
targets: [
{
src: 'package.json',
dest: 'dist',
transform: (contents) => {
const json = JSON.parse(contents.toString());
json.type = 'module'; // Add type: module
return JSON.stringify(json, null, 2);
}
}
]
})
]
};
// Our common output options, all configs will use these options
const commonOutputOptions: OutputOptions = {
sourcemap: true,
preserveModules: false // This ensures everything is bundled into one file
};
const config: RollupOptions[] = [
// Config for MJS output
// Used for modern browsers and bundlers
// import { DotCMSClient } from 'dotcms-client'
{
...commonInputOptions,
output: [
{
...commonOutputOptions,
file: `${outputPath}.mjs`,
format: 'es'
}
]
},
// Config for IIFE output
// Used for older browsers and bundlers
// to be imported on script tags
{
...commonInputOptions,
output: [
{
...commonOutputOptions,
name: 'dotcmsClient',
file: `${outputPath}.js`,
format: 'iife'
},
{
...commonOutputOptions,
name: 'dotcmsClient',
file: `${outputPath}.min.js`,
format: 'iife',
plugins: [terserPlugin()] // This plugin will minify our code, removing whitespace and other characters
}
]
},
// Config for CJS output
// Used for older bundlers like Browserify
// const DotCMSClient = require('dotcms-client')
{
...commonInputOptions,
output: [
{
...commonOutputOptions,
file: `${outputPath}.cjs`,
format: 'cjs'
}
]
},
// Config for TypeScript declaration output
// Used for TypeScript projects
// import { DotCMSClient } from 'dotcms-client'
{
input: 'src/index.ts',
plugins: [dtsPlugin()],
output: {
file: `${outputPath}.d.ts`,
format: 'es'
}
}
];
export default config;
I recommend taking a moment to read through the code carefully before we move forward. I’ve added comments to break it down for you.
Once this file is set up, we need to update tsconfig.json to include it in the include array. Modify it so it looks like this:
{
"compilerOptions": {
"esModuleInterop": true,
"skipLibCheck": true,
"target": "es2022",
"allowJs": true,
"resolveJsonModule": true,
"moduleDetection": "force",
"isolatedModules": true,
"strict": true,
"noUncheckedIndexedAccess": true,
"noImplicitOverride": true,
"module": "NodeNext",
"outDir": "dist",
"sourceMap": true,
"declaration": true,
"lib": ["es2022", "dom", "dom.iterable"]
},
"include": ["src/**/*", "rollup.config.ts"]
}
Now, let’s make a few changes on our package.json file:
{
"name": "dotcms-client",
"version": "1.0.0",
"main": "./dotcms-client.cjs",
"module": "./dotcms-client.mjs",
"types": "./dotcms-client.d.ts",
"scripts": {
"test": "echo \"Error: no test specified\" && exit 1",
"build": "rimraf dist && rollup --config rollup.config.ts --configPlugin @rollup/plugin-typescript --bundleConfigAsCjs"
},
"keywords": [],
"author": "",
"license": "ISC",
"description": "",
"exports": {
"./package.json": "./package.json",
".": {
"types": "./dotcms-client.d.ts",
"import": "./dotcms-client.mjs",
"require": "./dotcms-client.cjs",
"default": "./dotcms-client.mjs"
}
},
"typesVersions": {
"*": {
".": [
"./dotcms-client.d.ts"
]
}
},
"devDependencies": {
"@rollup/plugin-terser": "^0.4.4",
"@rollup/plugin-typescript": "^12.1.2",
"@types/node": "^22.13.13",
"rimraf": "^6.0.1",
"rollup": "^4.37.0",
"rollup-plugin-copy": "^3.5.0",
"rollup-plugin-dts": "^6.2.1",
"tslib": "^2.8.1",
"typescript": "^5.8.2"
}
}
The main changes are the addition of properties that help the Node module system locate the files:
main – Points to the CommonJS entry file.
module – Points to the ESM entry file.
types – Specifies the TypeScript type definitions file.
exports – Defines which files are exposed to consumers of your module.
typesVersions – Maps TypeScript versions to specific types.
We also added a new build script in package.json that looks like this:
"scripts": {
"build": "rimraf dist && rollup --config rollup.config.ts --configPlugin @rollup/plugin-typescript --bundleConfigAsCjs"
}
Breaking It Down
rimraf dist – Cleans up the dist folder before each build, ensuring that old build files are removed.
rollup --config rollup.config.ts – Tells Rollup to use the configuration we just set up in rollup.config.ts.
--configPlugin @rollup/plugin-typescript – Adds the TypeScript plugin to handle the TypeScript files.
--bundleConfigAsCjs – Bundles the configuration in CommonJS format, making it compatible with Node.js environments.
Before We Build
Let’s first modify our index.ts file to export the necessary components. Replace the file’s entire contents with this:
export { DotCMSClient } from './lib/client';
export type { DotCMSConfig, PageAPIParams } from './lib/types';
Once that’s done, we can build the project by running the following command:
npm run build
You should see some logs in the terminal showing the build process.
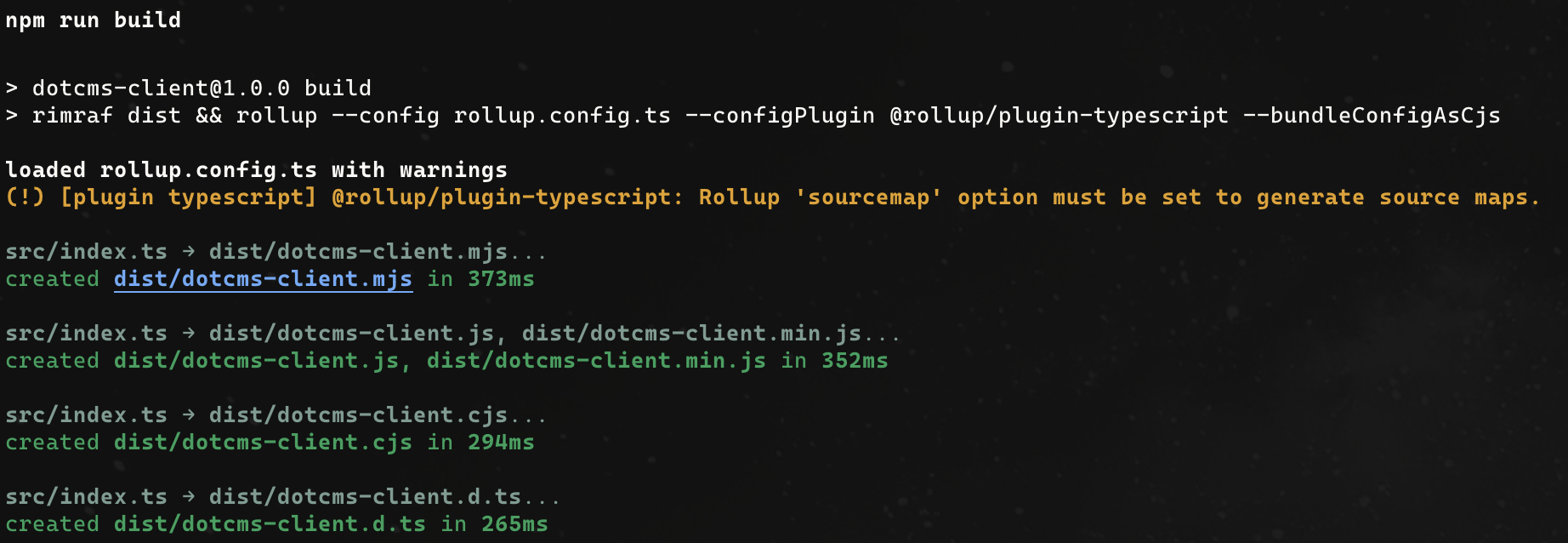
After the build completes, open your IDE and you’ll notice a new folder called dist. This folder will contain all the files we configured in Rollup.
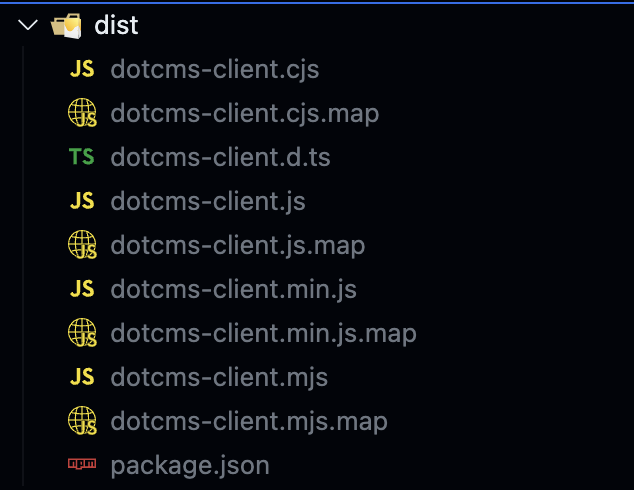
That means we’re now ready to publish our SDK!
Before We Publish
Let’s take a moment to review our package.json file. As you can see, there are some empty properties, like description, keywords, and author. These are there for you to fill in with your own information or relevant data that can help others find and understand your SDK. So, feel free to complete these before we continue.
Also, don’t forget to check the version property. You should update this version number every time you publish a new version of your SDK. For this example, I’m using 1.0.0, but here’s a helpful guide from NPM on how to manage versioning effectively: NPM Versioning Guide.
With that in mind, let’s first log in to NPM. Run the following command:
npm login
If you’re not already logged in, it will prompt you to open a specific link or hit enter. Follow the process, and once you’re successfully logged in, we need to publish our built code, so let’s move on to the dist folder:
cd dist
Finally, publish the SDK with:
npm publish
Once the process finishes, you can go to your NPM account to confirm that your code was successfully published. You can also try installing your SDK in an existing project by running:
npm i your-sdk-name
For example, using this SDK as an example:
npm i dotcms-client
Note: If you encounter any issues while publishing the SDK, try changing the name property in the package.json file. The SDK will be published under that name, and to install it afterward, you should use the command above with the name you chose.
Wrapping Up
And just like that, you now have an SDK that’s truly universal and easy to maintain, no matter the project or environment — no more headaches when reusing it.
You can find the complete code for this tutorial here, along with the published SDK.
Stay tuned for more updates and awesome tutorials coming your way! 🚀